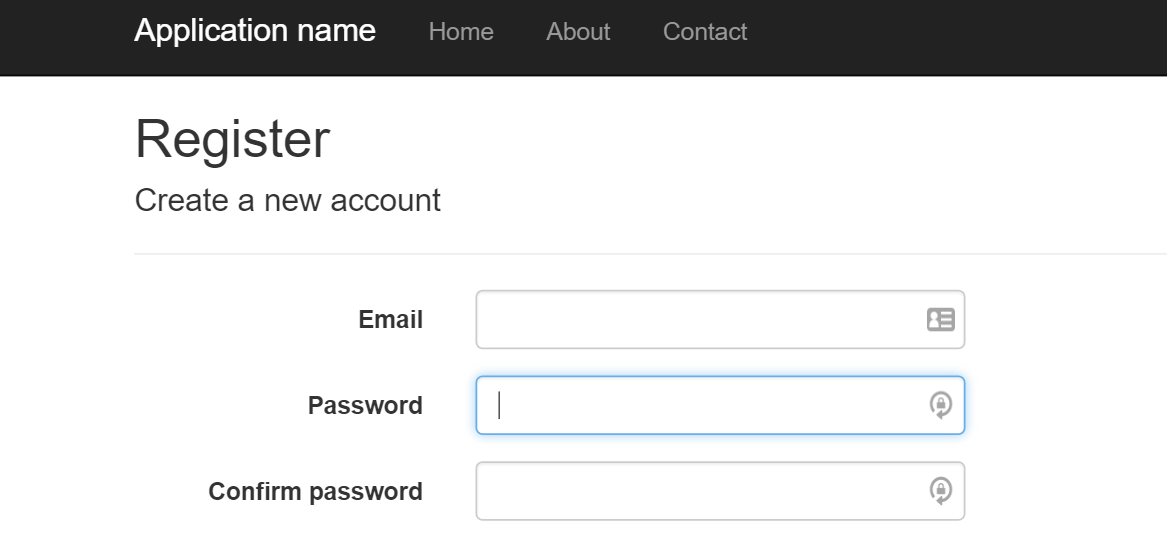
Setting up SendGrid 7.0.0 in ASP.NET
Today I was starting up a new ASP.NET Web Application using the ASP.NET Identity membership system, for a micro site I’m building, and my first task was to get the email confirmation working for accounts (and subsequently to prevent logging in until email has been confirmed). I decided to try out SendGrid rather than using my own SMTP server, mostly because, well, Microsoft told me I should 😉
In following the instructions on sending the email confirmation using the helpful tutorial Create a secure ASP.NET MVC 5 web app with log in, email confirmation and password reset, I discovered that the SendGrid API used in their sample code is an older version, and things have changed quite a bit in the SendGrid Web API version 7.0.0.
I recommend still following the above tutorial for the set up of the email confirmation sending in the ASP.NET application, but here’s the bit you’ll need to get it all going: an update to the ConfigSendGridAsync() function.
First you’ll now need an API key for SendGrid, so go to your SendGrid app (https://app.sendgrid.com), go to Settings > API Keys and click “Create API Key” at the top-right of the page to create a new one. I just selected “Mail Send” as the only permission to grant to this app, but you can customise however you see fit.
Now back in your app, I’ve chosen to store this API key in my web.config so that I can encrypt it later for production use, so in web.config I’ve added:
<add key="SendGrid_Key" value="SENDGRID_API_KEY_HERE" />
Finally, in IdentityConfig.cs (under /app_start), use the following for the helper class for configuring and sending via SendGrid:
private Task ConfigSendGridAsync(IdentityMessage message)
{
String apiKey = ConfigurationManager.AppSettings["SendGrid_Key"];
dynamic sg = new SendGridAPIClient(apiKey);
Email from = new Email("fromaddress@domain.coom");
String subject = message.Subject;
Email to = new Email(message.Destination);
Content content = new Content("text/plain", message.Body);
Mail mail = new Mail(from, subject, to, content);
Content htmlContent = new Content("text/html", message.Body);
mail.AddContent(htmlContent);
dynamic response = sg.client.mail.send.post(requestBody: mail.Get());
return Task.FromResult(0);
}
Note that I’m using the message that is passed in from the Identity SendAsync function to determine the recipient (destination) and body content, and I’m adding both a text and HTML version.
That’s it, now having followed all the other instructions in the original tutorial for turning on the email confirmation, my app is sending these via SendGrid (and all for free because it will be a low-traffic app).
The bonus of all this is that I’m not using my own SMTP server like I always used to, and SendGrid tracks all actions performed on emails that it sends, so I can have more visibility when I get the typical support ticket of “this customer says they didn’t get the confirmation email”. Neat!